Hi folks,
I'm trying to parse a binary string on a Red Lion DA30D using a Raw UDP/IP input port. I've done this before with ASCII strings so I do have some experience on the Red Lion, and I've got experience on parsing similar binary strings in Python.
But parsing a binary string in Red Lion gives me some headache. The data types to be parsed out is of different byte sizes and data types, please see attached message definition.
In addition to this, the payload has a wrapper around it, and I've been able to extract the message payload by identifying the start characters, see code. From there, i do not know how to parse bytes into (u)INT16/32/48, Float32, Bit16 data types - Can someone please help, any ideas are welcome and minor clues might help as well!
Message Wrapper

Message Payload
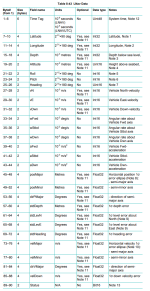
My start looks like this:
I'm trying to parse a binary string on a Red Lion DA30D using a Raw UDP/IP input port. I've done this before with ASCII strings so I do have some experience on the Red Lion, and I've got experience on parsing similar binary strings in Python.
But parsing a binary string in Red Lion gives me some headache. The data types to be parsed out is of different byte sizes and data types, please see attached message definition.
In addition to this, the payload has a wrapper around it, and I've been able to extract the message payload by identifying the start characters, see code. From there, i do not know how to parse bytes into (u)INT16/32/48, Float32, Bit16 data types - Can someone please help, any ideas are welcome and minor clues might help as well!
Message Wrapper

Message Payload
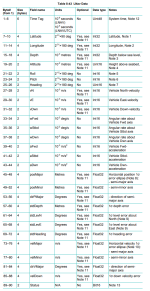
My start looks like this:
C#:
if (PortRead(7, 50) == 16) // HEX 10
{
if (PortRead(7, 50) == 2) // HEX 02
{
if (PortRead(7, 50) == 0) // HEX 00
{
if (PortRead(7, 50) == 224) // HEX E0
{
// MESSAGE IS 'LNAV' TYPE
// PAYLOAD FOLLOWS HERE
}
else if (PortRead(7, 50) == 232) // HEX E8
{
// MESSAGE IS 'LNAVUTC' TYPE
// PAYLOAD FOLLOWS HERE
}
}
}
}