I will try to explain how it might work when compiled
Assume you want to make a block (call it a block because it could be a function or function block depending what you need to do.
This block is a simple one i.e. an add (I know there will be an add function but bear with me).
so you have two variables A & B and you need a result C the block will require two input vars & one output.
So in the block it will contain the logic ADD A + B out to C (IN (A) IN (B) OUT (Result)
When you call the block with an instance call it ADD_FUNCTION
Assume your variables are called Var_1 & Var_2 these contain say 3 & 5
The output variable is called Var_3.
When you call the block the compiler will do something like this
Main program
.
.
//Note: A, B, Result are the parameter assignments for the block
Call ADD_Function // just to indicate you are calling ther function
Move Var_1 > A //moves the var into the block memory
Move Var_2 > B // moves the second var into the block memory
JUMP ADD_FUNCTION //Jumps past this main routine after the end of the main program see code later
Move Result > Var_3 // when the function returns it moves the result back to the variable.
... Rest of program
....
....
END // End of cyclic program
ADD_FUNCTION: // program jumps here
ADD A & B MOV Result // adds the vars & moves it to result
RET // returns back to main program.
END
If you call this block many more times with different variables but as the same instance it jumps to the same code, however, if you create each one as a seperate instance then the compiler produces multiple add functions after the end of the main program.
So for example
Main code
Call Add_Function_1 (instance 1 of the function)
Call Add_Function_2 (instance 2) & so on
.
.
.
END
Add_Function_1 (first instance)
.
.
.
RET
Add_Function_2 (second instance
.
.
.
RET
& so on for as many instances you create.
EDIT: forgot to mention that I have just a true contact in the call to the function but if you wanted to have many using the same variables i.e. at particular parts of the program then just use some logic to call the function when required in that EN pin.
Here is an example of calling the same instance more than once with different variables (Note: the FB instance is the same name so only one block of code is generated, if the names were different then there would be two blocks of code ).
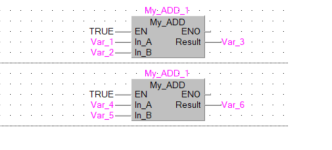